Local Notification
- 사용자 기기에서 발생하는 알림으로, 서버 없이 앱 내부에서 특정 이벤트 / 시간을 기반으로 알림 트리거 가능
- 인터넷 연결 없이도 동작하며, 앱 사용자에게 중요한 정보를 전달하는 데 유용함
로컬 알림의 주요 특징
1. 인터넷 연결 불필요
- 로컬 알림은 기기 내에서 트리거되므로 네트워크 연결 필요 없음
2. 앱 내부 이벤트 기반
- 특정 시간이나 이벤트에 따라 알림을 생성 가능
- 예를 들어, 특정 작업의 마감 알림이나 데일리 리마인더를 설정 가능
3. 제한된 컨트롤
- 앱이 백그라운드나 종료된 상태일 때는 알림만 표시 가능
로컬 알림 구현
(SwiftUI 기반으로 작성된 프로젝트입니다..!!)
1. 권한 요청
- 앱이 로컬 알림을 보내기 전에 사용자로부터 알림 권한 요청
import UserNotifications
final class NotificationManager {
static let shared = NotificationManager()
func requestNotificationPermission() {
let center = UNUserNotificationCenter.current()
center.requestAuthorization(options: [.alert, .sound, .badge]) { granted, error in
if let error = error {
print("권한 허용 에러: \(error.localizedDescription)")
}
print("권한 허용 승인: \(granted)")
}
}
}
- options는 알림에 표시할 요소(배너, 사운드, 배지)를 지정
2. 알림 생성 및 트리거 설정
- 특정 시간 또는 이벤트에 맞춰 알림 생성
func scheduleDailyNotification() {
let content = UNMutableNotificationContent()
content.title = "미라클 모닝"
content.body = "오늘 하루를 시작해보세요!"
content.sound = .default
// 매일 오전 5시에 알림
var dateComponents = DateComponents()
dateComponents.hour = 5
dateComponents.minute = 0
let trigger = UNCalendarNotificationTrigger(dateMatching: dateComponents, repeats: true)
let request = UNNotificationRequest(identifier: "dailyReminder", content: content, trigger: trigger)
let center = UNUserNotificationCenter.current()
center.add(request) { error in
if let error = error {
print("알림 설정 실패: \(error.localizedDescription)")
}
}
}
3. Foreground 알림 표시 설정
- iOS 기본 동작에서는 앱이 foreground 상태일 때 알림 표시 X
- 이를 해결하기 위해선 UNUserNotificationCenterDelegate 설정
class NotificationDelegate: NSObject, UNUserNotificationCenterDelegate {
func userNotificationCenter(
_ center: UNUserNotificationCenter,
willPresent notification: UNNotification,
withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void
) {
completionHandler([.banner, .sound])
}
}
- 설정 후, App delegate 에서 해당 delegate 설정
class AppDelegate: NSObject, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey : Any]? = nil) -> Bool {
UNUserNotificationCenter.current().delegate = NotificationDelegate()
return true
}
}
4. 알림 권한 요청 및 트리거
- 앱 실행 시, 알림 권한 요청 하고 알림 스케줄 등록
@main
struct YourApp: App {
init() {
NotificationManager.shared.requestNotificationPermission()
}
var body: some Scene {
WindowGroup {
ContentView()
.onAppear {
NotificationManager.shared.scheduleDailyNotification()
}
}
}
}
결과는..?
<권한 설정 알림>
<알림 화면>
<메인 화면>
성공!
.
.
.
.
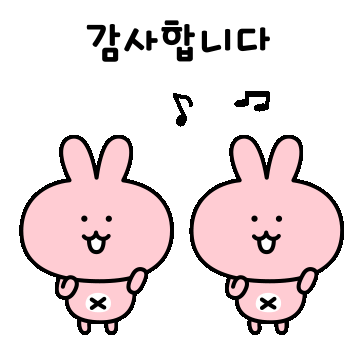
'Swift' 카테고리의 다른 글
[iOS] About Kingfisher (1) | 2024.11.27 |
---|---|
[iOS] About 채팅 UI (0) | 2024.11.20 |
[iOS] About 동시성 (0) | 2024.11.10 |
[iOS] About WidgetKit - 2(App Group) (0) | 2024.11.02 |
[iOS] About Notification & Delegation (0) | 2024.10.26 |